Think of a scenario that you have developed an e-commerce application using Flask version A. Year later, you want to develop another Python application using Flask, but this time you need to use version B, which has some new features that are not available in version A. However, when you try to upgrade to version B, you find that some features that were supported in version A are no longer supported in version B. This can be a frustrating situation, especially if you are using the system Python, which is common to all packages. In this case, you may find yourself in a deadlock, unable to use either version A or version B without encountering errors.
To avoid this problem, Python provides a feature called a virtual environment, which allows you to create isolated Python environments for different projects. With a virtual environment, you can install and use different versions of Flask (or any other library or package) for different projects, without worrying about conflicts or compatibility issues.
Benefits of using virtual environments for Python applications include:
- Isolation of dependencies: Each virtual environment has its own set of libraries and dependencies, which allows you to have separate environments for different projects without worrying about conflicts between library versions.
- Improved organization: Virtual environments allow you to keep your projects organized and separate, making it easier to find and work on specific projects.
- Ease of use: Virtual environments are easy to create and switch between, allowing you to quickly switch between different environments as needed.
Ways to create virtual environments for Python
There are several ways to create virtual environments for Python:
Using the built-in venv
module:
Python 3.3
and higher include a built-in venv module that allows you to create virtual environments. To create a virtual environment using venv
, use the following command:
python3 -m venv path/to/venv
Using the virtualenv package:
virtualenv
is a third-party package that allows you to create multiple Python environments. To install virtualenv
, use the following command:
pip install virtualenv
To create a virtual environment using virtualenv
, use the following command:
virtualenv path/to/venv
Activate the virtual environment by running the following command:
source venv/bin/activate
Your virtual environment is now active, and you can start installing packages and libraries specific to your project.
When you are finished working in the virtual environment and want to deactivate it, use the following command:
deactivate
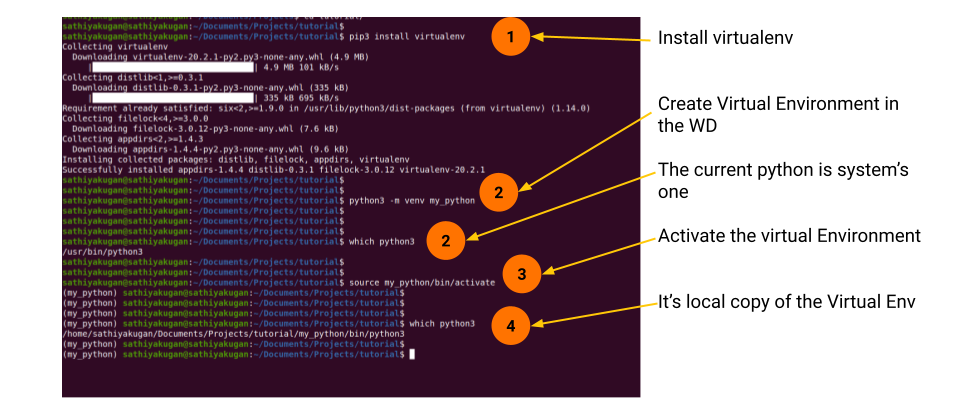
Using other tools:
There are other tools available for creating virtual environments, such as pyenv
and conda
. pyenv
is a tool that allows you to manage multiple Python versions and create virtual environments, while conda
is a package and environment management system that is commonly used in data science and scientific computing.
Conda
Conda does two things: manages packages and manages environments.
As a package manager, conda makes it easy to install Python packages, especially for data science. For instance, typing conda install numpy
installs the numpy
package.
As an environment manager, conda
allows you to create silo-ed Python installations. With an environment manager, you can install packages on your computer without affecting your main Python installation.
The command line code looks something like the following:
conda create --name environmentname
source activate environmentname
conda install numpy
Dockerizing Python applications
Docker is a tool that allows you to package an application and its dependencies in a single container, making it easy to deploy and run the application in any environment.
Benefits of using Docker for Python applications include:
- Portability: Docker containers can be run on any machine that has Docker installed, making it easy to deploy applications in different environments.
- Isolation: Docker containers allow you to isolate your application and its dependencies from the host system, ensuring that your application will run consistently across different environments.
- Ease of use: Docker makes it easy to package and deploy applications, making it a convenient tool for development and deployment.
Comparison of virtual environments and Docker
Virtual environments and Docker are similar in that they both allow you to isolate your application and its dependencies from the rest of the system. However, there are some key differences between the two:
- Scope: Virtual environments are used to isolate environments on a single machine, while Docker is used to package and deploy applications in different environments.
- Isolation: Virtual environments provide isolation at the Python level, while Docker provides isolation at the operating system level.
- Portability: Virtual environments are not portable, as they are specific to the machine they are created on. Docker containers, on the other hand, can be run on any machine with Docker installed.
In general, virtual environments are useful for isolating Python environments on a single machine, while Docker is useful for packaging and deploying applications in different environments
To dockerize a Python application, follow these steps:
- Install Docker on your machine.
- Create a
Dockerfile
in the root directory of your project. ADockerfile
is a text file that contains instructions for building a Docker image. - In the
Dockerfile
, specify the base image for your container. For Python applications, this will typically be a base image with a Python runtime. For example:FROM python:3.8
- Next, install any dependencies required by your application using the pip package manager. For example:
RUN pip install -r requirements.txt
- Add any additional files or configurations needed for your application.
- Set the working directory for the container and specify the command to run when the container is started. For example:
WORKDIR /app CMD python app.py
- Build the Docker image using the following command:
docker build -t my-app .
- Run the Docker container using the following command:
docker run -p 8080:8080 my-app
This will start the container and expose the application on port
8080
.
Conclusion
Virtual environments and Docker are useful tools for isolating and organizing Python environments and applications. Virtual environments allow you to create isolated Python environments on a single machine, while Docker allows you to package and deploy applications in different environments. Both tools have their own specific use cases and can be used together to improve the organization and portability of Python applications.
It is generally a good practice to use virtual environments for local development and testing, and to use Docker for deployment and production environments. By using these tools, you can ensure that your Python applications are isolated, organized, and portable, making them easier to develop and maintain.